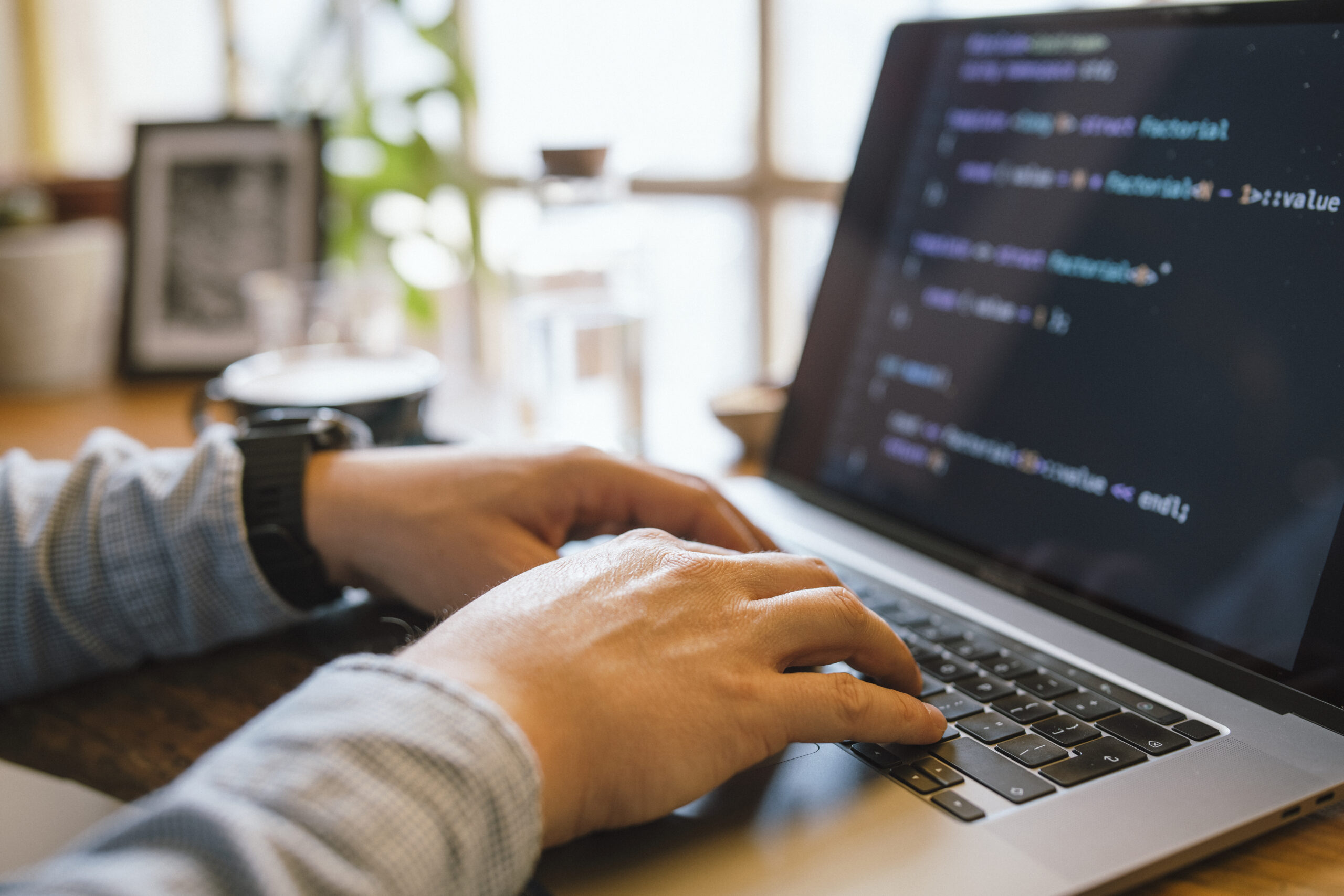
Debugging is Just about the most critical — but typically forgotten — skills inside a developer’s toolkit. It is not nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Discovering to think methodically to solve problems efficiently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can save hours of frustration and dramatically improve your efficiency. Here i will discuss various tactics to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
Among the list of quickest methods builders can elevate their debugging competencies is by mastering the instruments they use every single day. Although writing code is a person Component of growth, realizing how you can connect with it properly in the course of execution is equally significant. Modern day improvement environments occur Outfitted with powerful debugging abilities — but several builders only scratch the floor of what these applications can do.
Take, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, action by means of code line by line, and perhaps modify code about the fly. When used effectively, they let you notice exactly how your code behaves for the duration of execution, that is priceless for monitoring down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, monitor network requests, watch genuine-time effectiveness metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn aggravating UI challenges into manageable duties.
For backend or procedure-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Finding out these applications may have a steeper Understanding curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into snug with version Management methods like Git to comprehend code record, find the exact second bugs ended up released, and isolate problematic modifications.
Eventually, mastering your applications means heading further than default settings and shortcuts — it’s about producing an intimate understanding of your growth ecosystem in order that when challenges arise, you’re not misplaced at nighttime. The higher you already know your instruments, the greater time you are able to commit fixing the particular trouble rather than fumbling through the procedure.
Reproduce the Problem
Probably the most crucial — and often ignored — measures in powerful debugging is reproducing the challenge. Just before jumping into the code or earning guesses, builders need to have to create a consistent environment or state of affairs wherever the bug reliably appears. With out reproducibility, fixing a bug results in being a match of possibility, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a challenge is collecting just as much context as you can. Inquire questions like: What steps led to The difficulty? Which surroundings was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the precise circumstances less than which the bug happens.
As you’ve collected more than enough data, try to recreate the situation in your local setting. This could indicate inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge conditions or state transitions included. These checks not just support expose the problem but in addition protect against regressions in the future.
Often, The difficulty may be surroundings-unique — it might take place only on specified functioning methods, browsers, or below unique configurations. Applying resources like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It needs endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are presently halfway to repairing it. By using a reproducible circumstance, You may use your debugging applications extra effectively, test possible fixes safely, and communicate more clearly with your team or users. It turns an summary criticism right into a concrete problem — and that’s in which developers prosper.
Browse and Have an understanding of the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Incorrect. Instead of seeing them as disheartening interruptions, builders need to find out to treat mistake messages as immediate communications from your method. They often show you just what exactly took place, in which it happened, and in some cases even why it took place — if you know how to interpret them.
Start by looking at the concept carefully As well as in total. Numerous builders, particularly when under time force, glance at the main line and quickly begin earning assumptions. But deeper in the mistake stack or logs might lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — examine and realize them first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it position to a selected file and line variety? What module or function induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Studying to acknowledge these can greatly quicken your debugging approach.
Some faults are vague or generic, and in All those instances, it’s critical to look at the context in which the error transpired. Test related log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings either. These usually precede much larger issues and provide hints about prospective bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles a lot quicker, cut down debugging time, and turn into a more effective and assured developer.
Use Logging Properly
Logging is The most strong instruments in a very developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood while not having to pause execution or action from the code line by line.
A fantastic logging tactic commences with being aware of what to log and at what amount. Common logging levels include DEBUG, INFO, Alert, Mistake, and Lethal. Use DEBUG for thorough diagnostic data for the duration of advancement, Information for general events (like thriving start out-ups), WARN for possible issues that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure critical messages and slow down your procedure. Center on crucial events, point out adjustments, enter/output values, and significant choice details with your code.
Format your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in generation environments exactly where stepping by way of code isn’t possible.
Moreover, website use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a very well-thought-out logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the Total maintainability and trustworthiness of your code.
Believe Just like a Detective
Debugging is not simply a technological job—it's a kind of investigation. To proficiently identify and repair bugs, developers have to solution the procedure like a detective solving a thriller. This way of thinking allows break down complicated concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the symptoms of the issue: error messages, incorrect output, or efficiency concerns. Similar to a detective surveys a criminal offense scene, acquire as much pertinent details as you'll be able to with no jumping to conclusions. Use logs, check circumstances, and user reports to piece together a transparent photograph of what’s occurring.
Following, kind hypotheses. Question oneself: What could possibly be leading to this behavior? Have any changes a short while ago been designed to your codebase? Has this situation occurred before less than very similar situation? The aim would be to slender down options and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation within a managed natural environment. Should you suspect a specific purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and Enable the outcome lead you nearer to the truth.
Fork out close notice to modest details. Bugs generally hide from the least envisioned locations—similar to a missing semicolon, an off-by-just one error, or maybe a race situation. Be complete and affected person, resisting the urge to patch The difficulty with out thoroughly comprehending it. Momentary fixes might cover the actual difficulty, just for it to resurface later.
And finally, continue to keep notes on Everything you tried out and discovered. Just as detectives log their investigations, documenting your debugging course of action can preserve time for upcoming problems and support Many others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed challenges in complicated programs.
Produce Checks
Writing tests is one of the best solutions to help your debugging skills and General advancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety Web that gives you self-confidence when creating adjustments in your codebase. A properly-examined application is simpler to debug since it lets you pinpoint just wherever and when a challenge happens.
Begin with unit exams, which give attention to particular person capabilities or modules. These compact, isolated tests can quickly reveal regardless of whether a particular piece of logic is Operating as expected. When a exam fails, you straight away know wherever to seem, drastically lowering the time spent debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear right after previously remaining fastened.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These enable be certain that different parts of your software perform together effortlessly. They’re specifically helpful for catching bugs that manifest in intricate methods with various elements or services interacting. If a thing breaks, your exams can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To test a element correctly, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug might be a robust first step. When the exam fails constantly, you may concentrate on repairing the bug and enjoy your test move when The problem is fixed. This method makes sure that the exact same bug doesn’t return in the future.
In brief, composing checks turns debugging from the irritating guessing recreation right into a structured and predictable course of action—helping you catch a lot more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—staring at your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging applications is solely stepping absent. Having breaks allows you reset your intellect, reduce frustration, and often see the issue from the new standpoint.
If you're far too near the code for far too very long, cognitive tiredness sets in. You could possibly start off overlooking evident problems or misreading code that you just wrote just hrs earlier. Within this state, your Mind will become a lot less successful at dilemma-solving. A short wander, a espresso break, or perhaps switching to a different endeavor for ten–15 minutes can refresh your concentrate. Many builders report obtaining the basis of a problem when they've taken time and energy to disconnect, allowing their subconscious work during the qualifications.
Breaks also aid stop burnout, especially through more time debugging sessions. Sitting down in front of a screen, mentally caught, is not just unproductive but will also draining. Stepping absent enables you to return with renewed Electrical power plus a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular below restricted deadlines, but it in fact leads to more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a sensible strategy. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Discover From Just about every Bug
Every bug you experience is much more than simply A short lived setback—it's an opportunity to increase for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something beneficial in case you make the effort to replicate and review what went wrong.
Begin by asking oneself a number of critical issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit tests, code reviews, or logging? The responses normally expose blind places as part of your workflow or knowledge and assist you Make much better coding behaviors transferring forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see styles—recurring troubles or widespread blunders—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with all your friends could be Particularly impressive. No matter if it’s by way of a Slack message, a brief compose-up, or A fast know-how-sharing session, aiding others steer clear of the identical issue boosts staff efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial aspects of your advancement journey. After all, many of the very best builders aren't those who write best code, but those who continually learn from their blunders.
Eventually, Each and every bug you take care of adds a whole new layer to your skill established. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, additional able developer as a result of it.
Summary
Improving upon your debugging abilities can take time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.